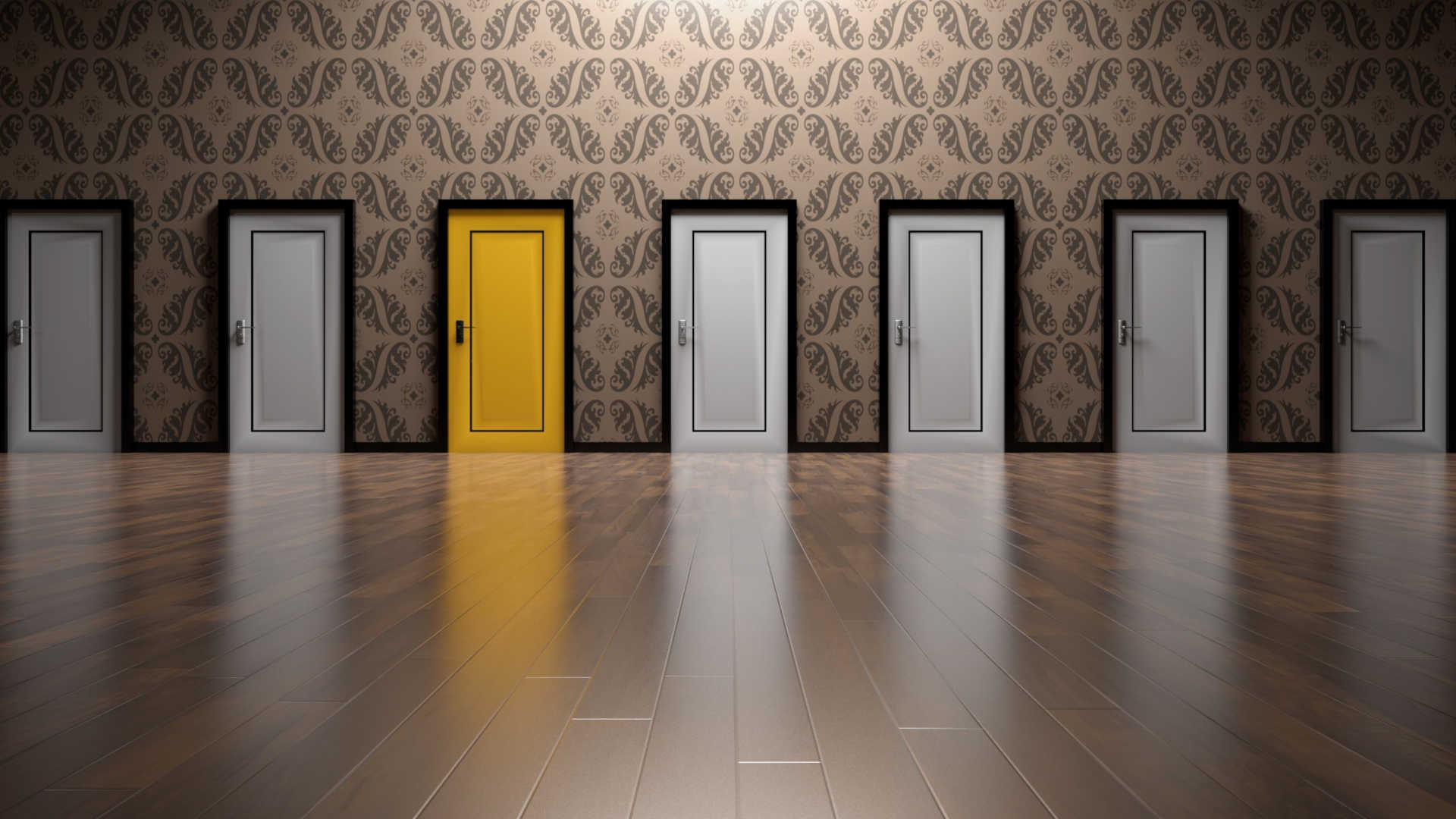
The Different Between Break, Return & Exit In PowerShell
This article explains the different between break, return and exit in PowerShell.
Question
What is the difference between the keywords Exit
, Return
and Break
in PowerShell?
What does each exactly do?
Short Answer
Break
terminates execution of a loop or switch statement and hands over control to next statement after it.Return
terminates execution of the current function and passes control to the statement immediately after the function call.Exit
terminates the current execution session altogether. It also closes the console window and may or may not close ISE depending on what direction the wind is facing.
Here are some examples…
Long Answer
Break
We use the Break
statement when we want to “jump” out of a For
, ForEach
, While
, Do
loop or Switch
statement and then continue execution immediately after that.
In this example, an infinite loop is broken when the iteration variable reaches the value 3:
$i = 1;
Write-Host "Starting the loop...";
while ($true)
{
Write-Host "Loop iteration $i";
if (($i += 1) -ge 3)
{
break; # exit the loop
}
}
Write-Host "Finished the loop!";
The example outputs:
Starting the loop...
Loop iteration 1
Loop iteration 2
Finished the loop!
As we can see, the loop was broken and execution continued right after it.
Return
We can use Return
statement when we want to jump out of a function and pass control to calling code. Execution then continues right after the function call itself.
Here’s our loop code again, this time wrapped up as a function:
# declare the function
Function Make-Pumpkins
{
$i = 1;
Write-Host "Making pumpkins...";
while ($true)
{
Write-Host "Pumpkin $i";
if (($i += 1) -ge 3)
{
return; # exit the function
}
}
Write-Host "Finished making pumpkins!";
}
# call the function
Write-Host "Calling the function...";
Make-Pumpkins;
Write-Host "Finished running the function!";
We have replaced the Break
statement with a Return
statement. Running the code now produces this:
Calling the function...
Making pumpkins...
Pumpkin 1
Pumpkin 2
Finished running the function!
Do you notice the difference? We no longer see that output at the end of the loop. Yet, we do see that execution continued right after the function call.
Exit
This is quite the oddball. The Exit
statement isn’t really a CmdLet to begin with. The Exit
statement is an internal command of PowerShell that instructs it to terminate the session altogether. Interestingly, this has slightly different effects, depending on what context and tool it is executed on.
Console: If we run this command straight on the PowerShell Console window, the window itself will close outright, no questions asked. It works just like the exit
command in the Command Prompt.
Script: If we run this command from a save script file, while on the console, it’s another matter.
For example, let’s take the previous block of code, replace the Return
statement with an Exit
statement and save it in a script file named Do-Stuff.ps1
;
# declare the function
Function Make-Pumpkins
{
$i = 1;
Write-Host "Making pumpkins...";
while ($true)
{
Write-Host "Pumpkin $i";
if (($i += 1) -ge 3)
{
exit # exit the session
}
}
Write-Host "Finished making pumpkins!";
}
# call the function
Write-Host "Calling the function...";
Make-Pumpkins;
Write-Host "Finished running the function!";
When we call this script from the Console, this is the result we get:
Calling the function...
Making pumpkins...
Pumpkin 1
Pumpkin 2
Notice how execution stopped outright when the Exit
statement was issued. However, the Console window stayed open just fine this time.
ISE: The ISE has some curious behaviour as well.
If we paste the previous code in an ISE window, save it as a script and then run it, the result is the same as when we run the script in the PowerShell Console. The same interrupted result appears. Yet, ISE stays open.
If we paste the same code in an ISE window and don’t save it before running it, ISE will prompt us to save the file with a Yes/No/Cancel message box. If we choose anything else than Cancel, ISE closes down.
Weird, innit?