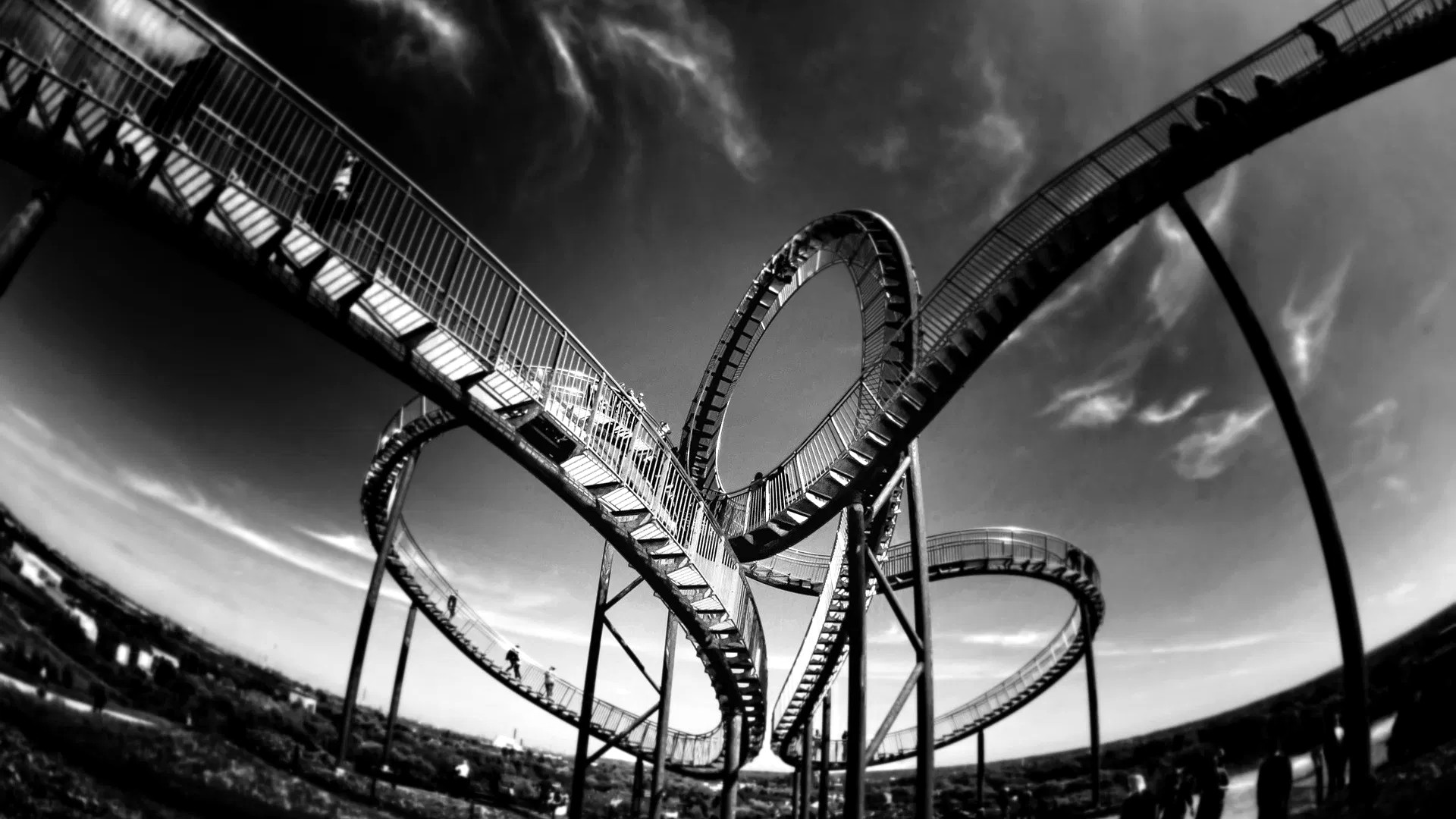
How To Loop And Index In PowerShell
Just learned these bits today while trying to answer something and thought to share them.
Consider these two arrays:
$x = @("a","b","c","d");
$y = @("apple","banana","carrot","dinner");
What I want to see as output is:
a is for apple
b is for banana
c is for carrot
d is for dinner
There are a couple of ways of going about this and then some.
You can use a regular for
loop:
for ($i = 0; $i -Lt $x.Count; ++$i)
{
"$($x[$i]) is for $($y[$i])";
}
You can go with a regular foreach
loop too this way, if the values in $x
are distinct - we’ll get around that in a second:
foreach ($v in $x)
{
"$v is for $($y[$x.IndexOf($v)])"
}
You can shorten it by using a ForEach-Object
CmdLet:
$x | ForEach-Object { "$_ is for $($y[$x.IndexOf($_)])" };
You can shorten the ForEach-Object
into the %
operator:
$x | % { "$_ is for $($y[$x.IndexOf($_)])" };
And finally, you can fix the issue of indexing duplicates by adding an initializer to the foreach and treating it just like a for
:
$x | % { $i = 0 } { "$_ is for $($y[$i])"; ++$i };
You learn something new every day.