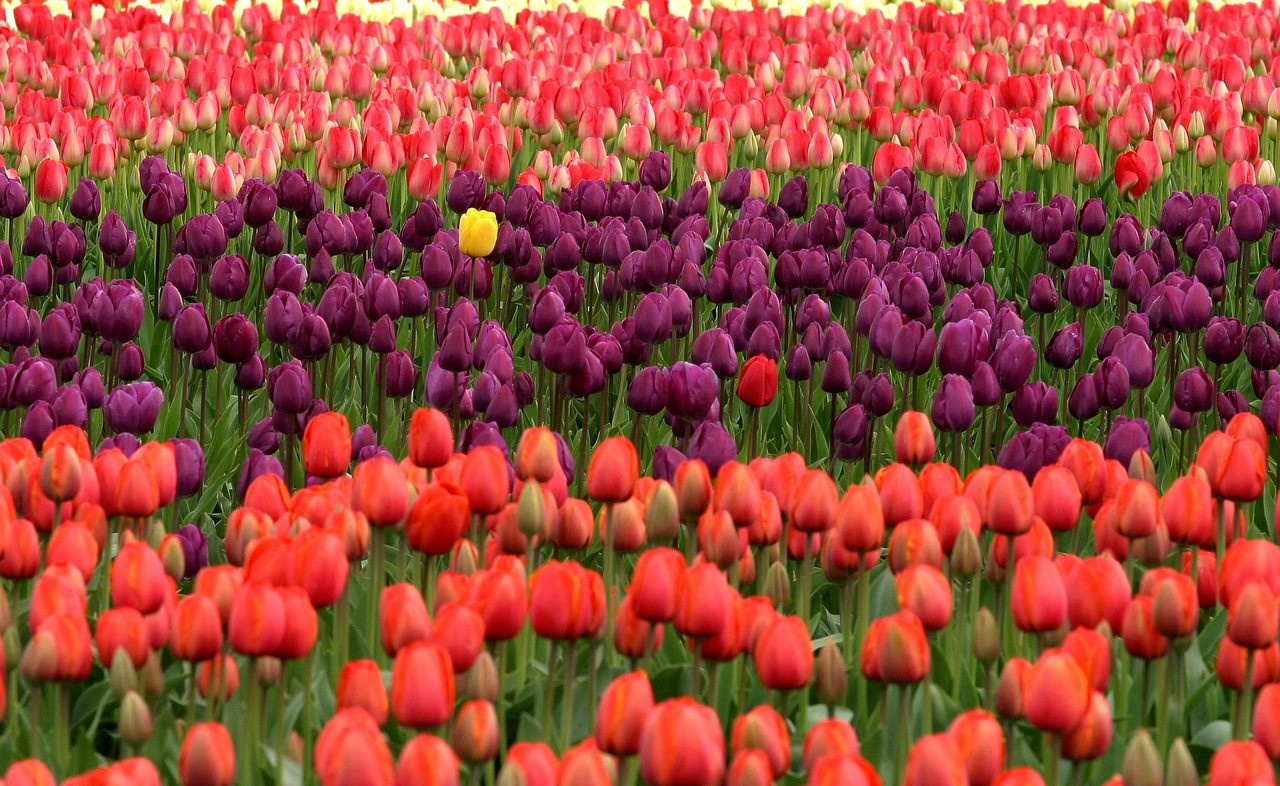
How to RegEx replace multiple strings in text files in PowerShell
What’s the only thing better than replacing multiple strings in text files using powershell? Why, doing it with regular expressions, of course! Here’s how.
Following from a previous post on doing a mass file replace with a lookup, the slightly changed snippet below will let you do it using the power of regular expressions to transform the values you replace along the way.
Param (
[String]$List = "TransformList.csv",
[String]$Files = ".Files*.txt"
)
$ReplacementList = Import-Csv $List;
Get-ChildItem $Files |
ForEach-Object {
$Content = Get-Content -Path $_.FullName;
foreach ($ReplacementItem in $ReplacementList)
{
$Content = $Content -IReplace $ReplacementItem.OldValue, $ReplacementItem.NewValue;
}
Set-Content -Path $_.FullName -Value $Content
}
Save this snippet as FindTransform-InFilesUsingLookup.ps1
in a folder of your choice.
You can run this script by either moving your files to the default locations, as noted by the $List
and $Files
parameters in the code, or, you can run this script as below:
.FindTransform-InFilesUsingLookup.ps1 .TransformList.csv .Files*.txt
Let’s give this a go. First of all, create a couple of files in the Files
folder (for lazyness sake) in the same folder as the script:
StuffToReplace1.txt
The quick brown fox jumps over the lazy dog over and over again
StuffToReplace2.txt
The funky dancing duck jumps over the lazy cat over and over again
Now create a list of transformations you wish to do. To keep it simple, I’ll only create one here. Our transformation will identify the subject and object in each text (fox
and dog
in the first one, duck
and cat
in the second one), and then switch them around.
Let’s take the text below and save it as TransformList.csv
in the same folder as the script:
OldValue,NewValue
(?<TheSubject>w+) jumps over the lazy (?<TheObject>w+),${TheObject} jumps over the lazy ${TheSubject}
Now execute the command below to see the results:
.FindTransform-InFilesUsingLookup.ps1 .TransformList.csv .Files*.txt
And this is what we get in the affected files:
StuffToReplace1.txt
The quick brown dog jumps over the lazy fox over and over again
StuffToReplace2.txt:
The funky dancing cat jumps over the lazy duck over and over again
Now this is what we call an inversion of roles - powered by PowerShell.